
Industry best practices for the MERN stack

There are a lot of different ways to build React apps and send them to production. One way is to build the React app with NodeJS and a database like MongoDB. This stack is popular for four reasons: you can write everything in JavaScript. MongoDB, React, Express, and NodeJS are the four things. This stack can be used in many different ways when building websites.
The whole front end is made with React, and MongoDB is used as a document database. The middle layer uses Express and NodeJS.
I conducted an interview with an industry professional who has experience working with the MERN stack. The purpose of that interview was to gain insights into industry best practices for the MERN stack, which was used to write this article.
In this article, I’ll talk about 10 best practices for making web applications with MERN. I’ll talk about things like organizing code, making sure it’s safe, and improving performance.
- If you’re developing a model in Node.js, Mongoose is the way to go.

Mongoose is an ODM library for MongoDB and Node.js that lets you model data using a schema-based approach to data modeling. This library streamlines the development process by offering pre-defined models that can be used to generate documents in MongoDB. This simplifies the management of data in MongoDB and reduces the amount of code required to build MERN-based apps.
Mongoose also helps keep the data correct by looking for mistakes before making changes to the database. This makes sure that only the correct information is stored, so mistakes or differences can’t happen. In addition, Mongoose enables connection definitions between collections, which simplifies the querying of related information.
2. Functional components are preferred over class components when working with React.

As compared to class components, which sometimes require the use of external libraries and sophisticated code, functional components are simpler and easier to comprehend. They’ll be easier to manage and less likely to have bugs as a result of this change.
Functional components can also be used for stateless components, which don’t store data locally but instead get it from a parent component’s callback. It’s better for performance and context separation if the component doesn’t have to be re-rendered when its props change. In addition to using functional components, developers may make use of React Hooks, which are a sophisticated means of handling state and side effects. By using hooks, developers can save time when putting things like local state management, lifecycle methods, and context in place. This simplifies testing and debugging while also keeping the codebase neat and tidy.
3. Use React’s Hooks to make your code more readable.
Because of the introduction of Hooks in React 16.8, developers are now able to take advantage of states and other React capabilities without having to create a custom class component. Because of this, the code is more simple, more comprehensible, and error-proof. With hooks, it’s simpler to reuse existing code and exchange logic across different components.
If you’re using Hooks, you’re probably already aware of how they may aid performance by preventing the rendering of components when they don’t need to be. When you use classes, you have to re-render the parent component even if the properties of the child components haven’t changed. With Hooks, we only have to re-render the parts of the page whose props have been modified.

Improvements in encapsulating functionality inside components are another benefit of using hooks. By separating common behaviors into custom hooks, developers can test new features quickly without having to make tests for the whole component. This aids in ensuring the integrity and bug-free nature of the code.
4. Use async/await in Node.js for managing asynchronous tasks.

Async/await lets programmers write asynchronous code that looks and works the same as synchronous code. Without being dependent on callbacks or promises, developers may produce code that is easier to read and understand. Using async/await makes it easier to find errors in asynchronous code because it processes them the same way it would process synchronous code.
There is potential for increased speed and scalability when async/await is used in connection with MERN. With async/await, programmers can wait until all pending tasks have finished before moving on to the next task. This guarantees that no unfinished business is ignored and that all available resources are put to good use. The async/await ability to process many requests in parallel has the potential to simultaneously lessen response times and boost throughput.
5. Take advantage of TypeScript’s static type checking and accelerated development speed.

TypeScript is a strongly typed extension of JavaScript that produces standard JavaScript upon compilation. It makes the language better by adding static typing as a replacement for dynamic typing and class-based object-oriented programming. Errors are caught early on, which helps cut down on development time. TypeScript also facilitates the management of large codebases by providing greater autocompletion and refactoring assistance than standard JavaScript.
Features like type inference, generics, and interfaces may all be used by developers working with TypeScript. These capabilities lessen the possibility of unexpected behavior caused by incompatible data types by maintaining a uniform format for all application data. Moreover, developers may use TypeScript to generate type definitions for third-party libraries, enabling them to be utilized without worrying about type compatibility concerns.
6. Use all the advanced capabilities, middleware, and routing that Express has to offer.
Express applications may greatly benefit from the use of middleware functionalities. You can use them to do things like login and authenticate, as well as make change requests and get answers. Built-in methods of middleware can be used to do a lot of different things, like validate user input, set response headers, and handle errors. With the help of middleware features, developers can quickly and easily add new features to their software.

Another crucial MERN best practice is the use of routing. Because of routing, programmers may specify how each URL should be processed. This simplifies the development of advanced web apps with many different sections and functions. With routing, programmers can easily make dynamic web pages by telling the server which parts to show based on the URL.
7. Use the data manipulation features of MongoDB’s Aggregation Framework.
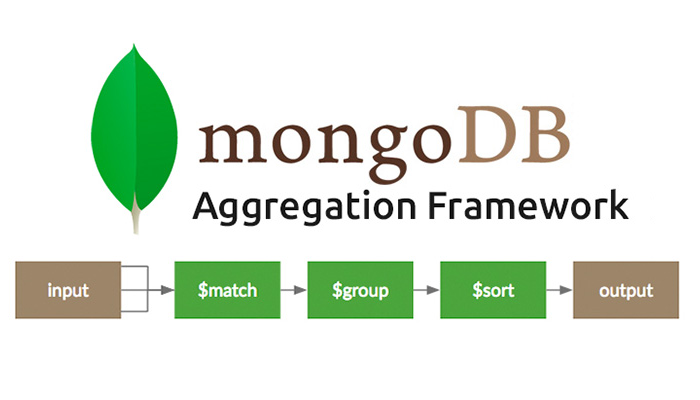
Developers may execute complicated operations on their data in a manner that is both efficient and scalable with the help of the Aggregation Framework. It has several operators for sorting, filtering, grouping, and limiting the results of a query, such as $match, $project, $group, $sort, and $limit. As a result, complex queries may be built quickly and with little effort.
Using the Aggregation Framework, you may potentially reduce network traffic and improve response times. The framework’s pipeline operators allow developers to restrict the number of documents returned by a query, reducing the amount of information sent between the server and the client. Since it is installed on the backend of the database server, the Aggregation Framework may make use of indexes and other server-side optimizations to speed up the processing of queries.
8. You may do more effective database queries by using an ORM such as Sequelize or Objection.js.
Since ORMs provide a buffer between the app and the database, programmers may use a language they’re already comfortable with (like JavaScript) instead of learning SQL. So, programmers can connect to databases with less work and without having to learn complicated SQL syntax. ORMs also make it simple for programmers to move their code from one database to another, like MongoDB or MySQL, without rewriting their queries.
Reducing the number of database calls required to obtain data is one way in which using an ORM like Sequelize or Objection.js may increase speed. While using an ORM, developers may avoid writing repetitive database queries. Object-relational managers (ORMs) also have useful features like validation, associations, and migrations that make data management easier.
9. Implement safe programming with the help of ESLint, Prettier, and Flow.

ESLint is a tool for static code analysis that may be used by programmers to find and repair vulnerabilities in their code. Common mistakes in coding, like using functions or variables that aren’t secure, can be found and flagged, so the developer can fix the problem before putting the code into production.
If you want your code to look the same throughout, use Prettier, an opinionated code formatter. The potential for security flaws as a result of miscommunication between developers is lessened when they are able to read and comprehend each other’s code.
Being a static type checker, Flow aids programmers in spotting type-related issues at an early stage. The earlier these issues are found and fixed, the less likely it is that additional severe security flaws will be introduced.
10. Use test automation frameworks like Jest, Enzyme, and Mocha.

Automated testing is a fantastic tool for making sure your code does what it’s supposed to and finding errors before they have a chance to slow you down. Frameworks like Jest, Enzyme, and Mocha make it easy for developers to automate the testing of backend services like Node.js servers and MongoDB databases and their frontend components like React.
The Jest testing framework was developed in JavaScript with React apps in mind. It facilitates early problem detection by enabling developers to construct unit tests for specific components. For testing React components, Enzyme is another popular package for simulating user interactions. This facilitates the testing of a component’s right interaction behavior. Last but not least, Mocha is a feature-rich JavaScript testing framework that can be used to test both client and server-side applications.
Automated testing may be made more effective and thorough by including these three frameworks in your MERN stack. Using these resources, developers can simply and rapidly test both the front- and back-end components of their software. By doing so, you may be certain that the code you’ve developed is completely stable and ready for deployment.
Conclusion
In conclusion, the MERN stack is a popular set of technologies for developing online applications. Using industry best practices may help developers create applications that are efficient, scalable, and easy to maintain. By adhering to standards for things like error handling and debugging, performance optimization, and scalability, developers can ensure their apps are robust and able to handle a large influx of users and data without slowing down. By following these guidelines, developers may create high-quality apps that help the company achieve its objectives.
Follow me on GitHub: Madhusha Prasad